之前研究过Memtest86+的编译,但是没有搞清楚为什么我自己编译出来的DOS可执行程序版本的始终无法运行,与之相对,编译出来的ISO版本或者软盘版都是正常的。经过一番研究,终于找到了问题的原因。在编译之前,需要修改mt86+_loader.asm中的一个定义:
%define fullsize (164504 + buffer – exeh)
; 164504 is the size of memtest86+ V4.20, adjust as needed!
其中这个164504 应该是你编译一次之后memtest.bin文件的大小。这个文件的大小会跟着你使用的gcc编译器版本不同或者其他原因有着不同的大小(万幸,每次编译结果大小是不变的)。在我的环境下,编译出来的memtest.bin大小为176760bytes,因此这个值需要重新修改。
简单解释,这个值是最终生成DOS EXE的文件头,它决定 Load到内存中的有用的文件代码的大小,当这个值偏小(实际有用的代码要更多,装入的少),会导致不可预料的后果。这就是为什么之前编译出来的并不稳定的原因。
顺便说两句
1. 不要尝试在Windows 7 的 XP Mode中安装Ubuntu 12.04,安装过程中会提示无法找到硬盘。推荐使用VirtualBox作为编译环境以及测试的虚拟机。在它上面能够正常运行的话,
在实体机上也不会有问题。
2. 特别注意,修改上述文件之后最好比较一下是否完全编译进入EXE中,最简单的方式是比较新生成的EXE和之前的EXE文件前面310h字节是否相同
3. Ubuntu 12.04没有内置Nasm,安装方法可以参考这篇 insnasm 文章,来自 Linux公社
4. 此外,还提供了一个包含我编译之后的exe和原始mt420.exe的iso镜像以供比较 mtlabz
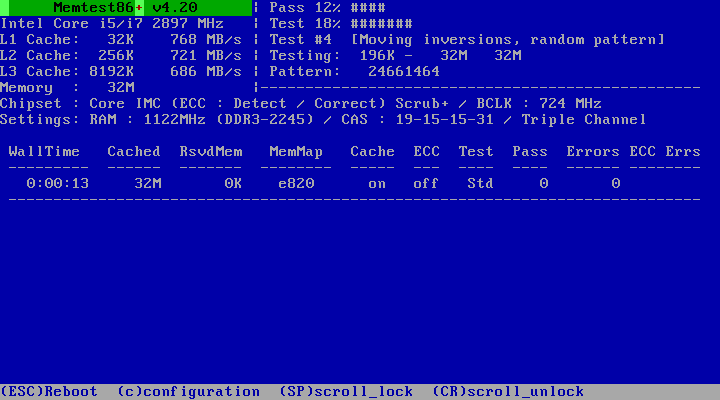
English Version
A. Source code is downloaded from
http://www.memtest.org/
or you can download a 4.20 here memtest86+-4.20.tar
B. Compiling environment
Ubuntu 12.04 can be downloaded from http://forum.ubuntu.org.cn/viewtopic.php?f=49&t=349550
Nasm can be downloaded from http://www.nasm.us/pub/nasm/releasebuilds/
C. Virtual machine: I use Virtual Box this time. And I have tested the ‘XP mode’ of Windows 7 it fails. I can install the Ubuntu to it. The Ubuntu 12.04 can’t find the HD on it(I use 12.04. And I’m not sure if other version works well). So please don’t’ use XP mode. It will drive you mad.
D. Install the Ubuntu in your Virtual Box. And you should install a Nasm. Ubuntu 12.04 doesn’t contain a nasm 🙁
E. Here is the key-point. You should modify the definiation in mt86+_loader.asm:
%define fullsize (164504 + buffer – exeh)
; 164504 is the size of memtest86+ V4.20, adjust as needed!
164504 is the size of memtest.bin. You run ‘make dos’ once and get the size of memtest.bin This value may be different if the gcc version is different. It reads 176760 bytes in my side.
F. At last build the source again with ‘make dos’. You will get a ‘memtest.exe’. It’s the a DOS executable file.
Note: Be sure to recompile mt86+_loader.asm! Compare the exe you get and the original one. The value in it head should be different.